Build HTML5 Games in JavaScript
Build HTML5 Games in JavaScript
{Firstname}, {Lastname}, {Conference}, {Location}, {date}
- Create the Canvas and draw on it
- Move the ball
- Bounce off the walls
- Paddle and keyboard controls
- Game over
- Build the brick field
- Collision detection
- Track the score and win
- Mouse controls
- Finishing up
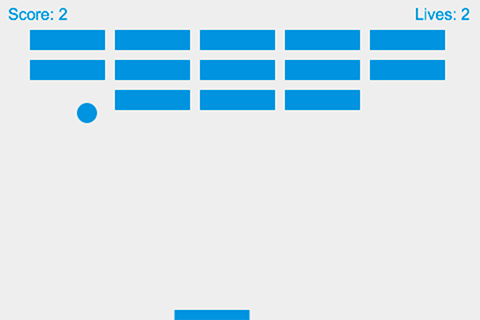
- Basic JavaScript knowledge
- Open mind, eager to learn
1. Create the Canvas and draw on it
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Gamedev Canvas Workshop</title>
<style>
* { padding: 0; margin: 0; }
canvas { background: #eee; display: block; margin: 0 auto; }
</style>
</head>
<body>
<canvas id="myCanvas" width="480" height="320"></canvas>
<script>
// JavaScript code goes here
</script>
</body>
</html>
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.beginPath();
ctx.rect(20, 40, 50, 50);
ctx.fillStyle = "#FF0000";
ctx.fill();
ctx.closePath();
ctx.beginPath();
ctx.arc(240, 160, 20, 0, Math.PI*2, false);
ctx.fillStyle = "green";
ctx.fill();
ctx.closePath();
ctx.beginPath();
ctx.rect(160, 10, 100, 40);
ctx.strokeStyle = "rgba(0, 0, 255, 0.5)";
ctx.stroke();
ctx.closePath();
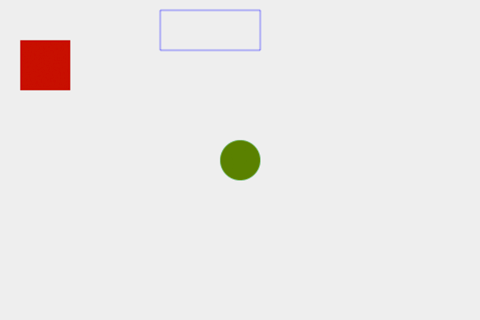
Change the size and color of the given shapes.
function draw() {
// drawing code
}
setInterval(draw, 10);
ctx.beginPath();
ctx.arc(50, 50, 10, 0, Math.PI*2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
var x = canvas.width/2;
var y = canvas.height-30;
Variables instead of constants
function draw() {
ctx.beginPath();
ctx.arc(x, y, 10, 0, Math.PI*2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
var dx = 2;
var dy = -2;
function draw() {
ctx.beginPath();
ctx.arc(x, y, 10, 0, Math.PI*2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
x += dx;
y += dy;
}
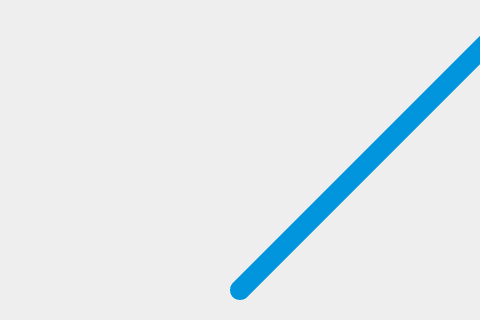
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.beginPath();
ctx.arc(x, y, 10, 0, Math.PI*2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
x += dx;
y += dy;
}
function drawBall() {
ctx.beginPath();
ctx.arc(x, y, 10, 0, Math.PI*2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBall();
x += dx;
y += dy;
}
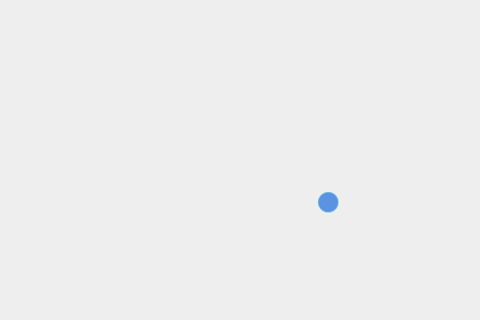
Change the speed of the moving ball, or the direction it moves in.
Simple collision detection
var ballRadius = 10;
ctx.arc(x, y, ballRadius, 0, Math.PI*2);
if(y + dy < 0) {
dy = -dy;
}
Bouncing off - the bottom
if(y + dy > canvas.height) {
dy = -dy;
}
Bouncing off the top and bottom
if(y + dy > canvas.height || y + dy < 0) {
dy = -dy;
}
Bouncing off all four directions
if(x + dx > canvas.width || x + dx < 0) {
dx = -dx;
}
if(y + dy > canvas.height || y + dy < 0) {
dy = -dy;
}
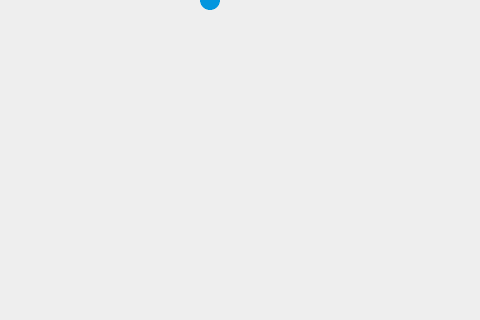
if(x + dx > canvas.width-ballRadius || x + dx < ballRadius) {
dx = -dx;
}
if(y + dy > canvas.height-ballRadius || y + dy < ballRadius) {
dy = -dy;
}
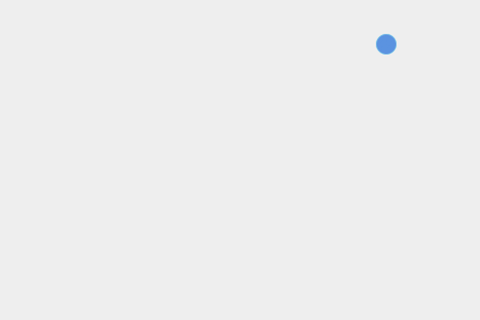
Change the color of the ball to a random colour every time it hits the wall.
4. Paddle and keyboard controls
var paddleHeight = 10;
var paddleWidth = 75;
var paddleX = (canvas.width-paddleWidth)/2;
function drawPaddle() {
ctx.beginPath();
ctx.rect(paddleX, canvas.height-paddleHeight,
paddleWidth, paddleHeight);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
How to control the paddle
- Two variables for left and right button presses
- Two event listeners for
keydown
and keyup
events
- Two functions handling the
keydown
and keyup
events
- Ability to actually move the paddle left and right
Variables storing key press
var rightPressed = false;
var leftPressed = false;
document.addEventListener("keydown", keyDownHandler, false);
document.addEventListener("keyup", keyUpHandler, false);
function keyDownHandler(e) {
if(e.keyCode == 39) {
rightPressed = true;
}
else if(e.keyCode == 37) {
leftPressed = true;
}
}
function keyUpHandler(e) {
if(e.keyCode == 39) {
rightPressed = false;
}
else if(e.keyCode == 37) {
leftPressed = false;
}
}
if(rightPressed) {
paddleX += 7;
}
else if(leftPressed) {
paddleX -= 7;
}
if(rightPressed && paddleX < canvas.width-paddleWidth) {
paddleX += 7;
}
else if(leftPressed && paddleX > 0) {
paddleX -= 7;
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBall();
drawPaddle();
}
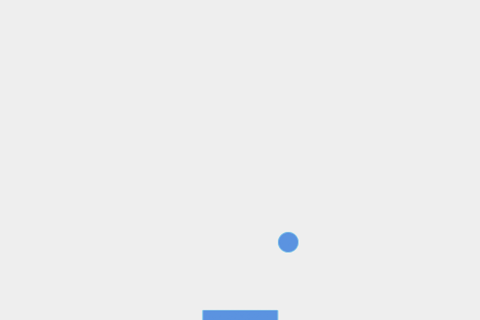
Make the paddle move faster or slower, or change its size.
if(x + dx > canvas.width-ballRadius || x + dx < ballRadius) {
dx = -dx;
}
if(y + dy > canvas.height-ballRadius || y + dy < ballRadius) {
dy = -dy;
}
if(x + dx < ballRadius) {
dx = -dx;
} else if(x + dx > canvas.width-ballRadius) {
dx = -dx;
}
if(y + dy < ballRadius) {
dy = -dy;
} else if(y + dy > canvas.height-ballRadius) {
alert("GAME OVER");
document.location.reload();
}
Hit the ball with the paddle
if(y + dy < ballRadius) {
dy = -dy;
} else if(y + dy > canvas.height-ballRadius) {
if(x > paddleX && x < paddleX + paddleWidth) {
dy = -dy;
}
else {
alert("GAME OVER");
document.location.reload();
}
}
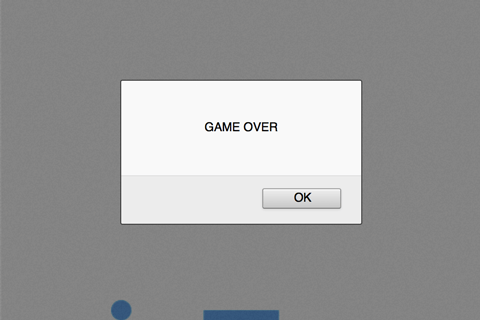
Make the ball move faster when it hits the paddle.
var brickRowCount = 5;
var brickColumnCount = 3;
var brickWidth = 75;
var brickHeight = 20;
var brickPadding = 10;
var brickOffsetTop = 30;
var brickOffsetLeft = 30;
var bricks = [];
for(c=0; c<brickColumnCount; c++) {
bricks[c] = [];
for(r=0; r<brickRowCount; r++) {
bricks[c][r] = { x: 0, y: 0 };
}
}
function drawBricks() {
for(c=0; c<brickColumnCount; c++) {
for(r=0; r<brickRowCount; r++) {
bricks[c][r].x = 0;
bricks[c][r].y = 0;
ctx.beginPath();
ctx.rect(0, 0, brickWidth, brickHeight);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
}
}
var brickX = (r*(brickWidth+brickPadding))+brickOffsetLeft;
var brickY = (c*(brickHeight+brickPadding))+brickOffsetTop;
function drawBricks() {
for(c=0; c<brickColumnCount; c++) {
for(r=0; r<brickRowCount; r++) {
var brickX = (r*(brickWidth+brickPadding))+brickOffsetLeft;
var brickY = (c*(brickHeight+brickPadding))+brickOffsetTop;
bricks[c][r].x = brickX;
bricks[c][r].y = brickY;
ctx.beginPath();
ctx.rect(brickX, brickY, brickWidth, brickHeight);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
}
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBricks();
drawBall();
drawPaddle();
}
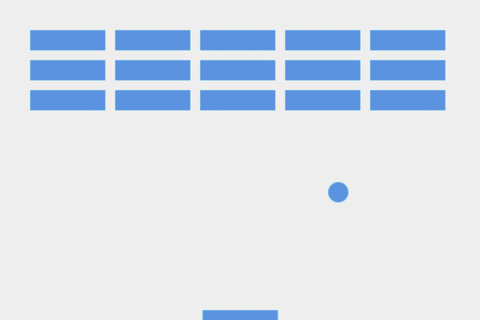
Change the number of bricks in a row or a column, or their positions.
Collision detection function
function collisionDetection() {
for(c=0; c<brickColumnCount; c++) {
for(r=0; r<brickRowCount; r++) {
var b = bricks[c][r];
// calculations
}
}
}
- The x position of the ball is greater than the x position of the brick.
- The x position of the ball is less than the x position of the brick minus its width.
- The y position of the ball is greater than the y position of the brick.
- The y position of the ball is less than the y position of the brick minus its width.
function collisionDetection() {
for(c=0; c<brickColumnCount; c++) {
for(r=0; r<brickRowCount; r++) {
var b = bricks[c][r];
if(x > b.x && x < b.x+brickWidth &&
y > b.y && y < b.y+brickHeight) {
dy = -dy;
}
}
}
}
var bricks = [];
for(c=0; c<brickColumnCount; c++) {
bricks[c] = [];
for(r=0; r<brickRowCount; r++) {
bricks[c][r] = { x: 0, y: 0, status: 1 };
}
}
function drawBricks() {
for(c=0; c<brickColumnCount; c++) {
for(r=0; r<brickRowCount; r++) {
if(bricks[c][r].status == 1) {
var brickX = (r*(brickWidth+brickPadding))+brickOffsetLeft;
var brickY = (c*(brickHeight+brickPadding))+brickOffsetTop;
bricks[c][r].x = brickX;
bricks[c][r].y = brickY;
ctx.beginPath();
ctx.rect(brickX, brickY, brickWidth, brickHeight);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
}
}
}
function collisionDetection() {
for(c=0; c<brickColumnCount; c++) {
for(r=0; r<brickRowCount; r++) {
var b = bricks[c][r];
if(b.status == 1) {
if(x > b.x && x < b.x+brickWidth &&
y > b.y && y < b.y+brickHeight) {
dy = -dy;
b.status = 0;
}
}
}
}
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBricks();
drawBall();
drawPaddle();
collisionDetection();
}
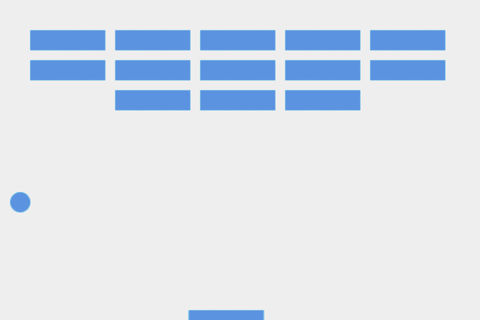
Change the color of the ball when it hits the brick.
8. Track the score and win
var score = 0;
function drawScore() {
ctx.font = "16px Arial";
ctx.fillStyle = "#0095DD";
ctx.fillText("Score: "+score, 8, 20);
}
function collisionDetection() {
for(c=0; c<brickColumnCount; c++) {
for(r=0; r<brickRowCount; r++) {
var b = bricks[c][r];
if(x > b.x && x < b.x+brickWidth &&
y > b.y && y < b.y+brickHeight) {
dy = -dy;
b.status = 0;
score++;
}
}
}
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBricks();
drawBall();
drawPaddle();
collisionDetection();
drawScore();
}
function collisionDetection() {
for(c=0; c<brickColumnCount; c++) {
for(r=0; r<brickRowCount; r++) {
var b = bricks[c][r];
if(x > b.x && x < b.x+brickWidth &&
y > b.y && y < b.y+brickHeight) {
dy = -dy;
b.status = 0;
score++;
if(score == brickRowCount*brickColumnCount) {
alert("YOU WIN, CONGRATULATIONS!");
document.location.reload();
}
}
}
}
}
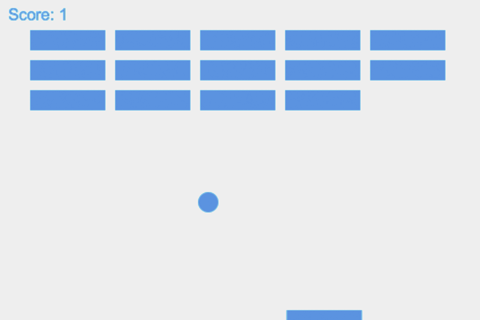
Add more points per brick hit, print out the number of collected points in the end game alert box.
document.addEventListener("mousemove", mouseMoveHandler, false);
function mouseMoveHandler(e) {
var relativeX = e.clientX - canvas.offsetLeft;
if(relativeX > 0 && relativeX < canvas.width) {
paddleX = relativeX - paddleWidth/2;
}
}
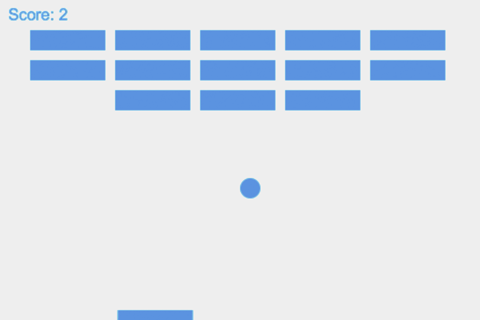
Adjust the boundaries of the paddle movement, so the whole paddle will be visible on both edges of the Canvas instead of only half of it.
var lives = 3;
function drawLives() {
ctx.font = "16px Arial";
ctx.fillStyle = "#0095DD";
ctx.fillText("Lives: "+lives, canvas.width-65, 20);
}
Removing life instead of game over
lives--;
if(!lives) {
alert("GAME OVER");
document.location.reload();
}
else {
x = canvas.width/2;
y = canvas.height-30;
dx = 2;
dy = -2;
paddleX = (canvas.width-paddleWidth)/2;
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBricks();
drawBall();
drawPaddle();
collisionDetection();
drawScore();
drawLives();
}
setInterval(draw, 10);
=> draw();
requestAnimationFrame(draw);
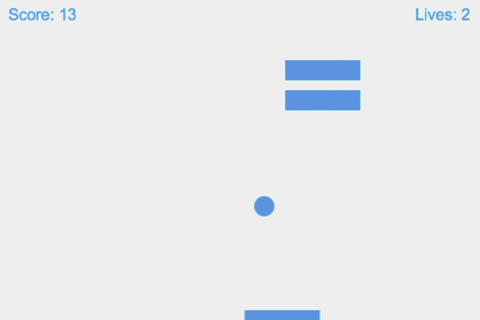
Change the number of lives and the angle the ball bounces off the paddle.
- {Your name and details}
- Slides: {slideurl}